Chaos17 Chevalier (niveau 2)
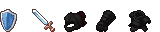

 Messages postés : 90 Date d'inscription : 09/02/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: [XP - ACE] Battle camera Ven 1 Mar 2013 - 23:18 | |
| Auteur : Ocedic Langue : anglais Catégorie : combat – addon Démo : non Site : rpg maker web Addon permettant d'avoir un effet de caméra qui bouge en même temps que vous taper un ennemi. Conversion d'un ancien script pour Rpg maker Vx Ace. EDIT : marche avec les SBS sans le motion battler de Moghunter. Script Vx Ace - Code:
-
# ***************************************************************************** # # OC BATTLE CAMERA # Author: Ocedic, KMS # Site: http://ocedic.wordpress.com/ # Version: 1.3 # Last Updated: 3/2/13 # # Updates: # 1.3 - Resolution support is now automatic, sprite y-axis adjustment is now # implemented, added compatibility with Victor Animated Battlers # 1.2 - Added new configuration settings # 1.1 - Removed some extraneous code, sprites now move based on camera target # 1.0 - First release # # *****************************************************************************
$imported = {} if $imported.nil?
#============================================================================== # ▼ Introduction # =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-= # This is an adaptation of KGC's Motion Battle Camera, which adds a moving # camera that follows the flow of battle. I used a lot of code by KGC, but # made my own modifications to get it working in Ace. #============================================================================== # ▼ Instructions # =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-= # Plug and play script. Simply paste it above Main. Settings can be adjusted # in configuration section. #============================================================================== # ▼ Compatibility # =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-= # This script works with YEA Battle System and Victor's Animated Battlers. It # also works with Jet's Viewed Battle System, but you'll have to enable the # SIDEVIEW_SYSTEM switch in configuration. Other SBSs may work depending on # their implementation. # Just make sure that it's placed below them. #============================================================================== # ▼ Terms of Use # =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-= # Free for non-commercial use. Credit must be given to both Ocedic and KMS. # This script is not for commercial use. #==============================================================================
#============================================================================== # ■ Configuration #------------------------------------------------------------------------------ # Change customizable settings here #============================================================================== module OC module OBC
#============================================================================== # * Camera Move Speed * #------------------------------------------------------------------------------ # Adjusts the camera speed, higher value is faster #============================================================================== CAMERA_SPEED_INIT = 24 #============================================================================== # * Sideview Battle System * #------------------------------------------------------------------------------ # Currently, only a handful of sideview battle systems are support (see above) # If you're using a support SBS, it should work automatically. If you're using # a different SBS, try setting this to true and it may work depending on how # the system is implemented. #============================================================================== # * Sideview: Move Actor Sprite Y-Axis * #------------------------------------------------------------------------------ # When using a SBS, choose whether to move the actor's sprite along the Y-axis # relative to the camera. Set it to false if you wish to disable this behavior # which may look weird on some systems like Jet's Viewed Battle. #============================================================================== SIDEVIEW_SYSTEM = false SBS_ACTOR_SPRITE_Y = true end end
#============================================================================== # ■ Game_Enemy #==============================================================================
class Game_Enemy < Game_Battler #============================================================================== # * Accessors * #============================================================================== attr_accessor :origin_x, :origin_y #============================================================================== # * Alias: Initialize * #============================================================================== alias oc_game_enemy_initialize_naslo initialize def initialize(index, enemy_id) oc_game_enemy_initialize_naslo(index, enemy_id) @origin_x = 0 @origin_y = 0 end
#============================================================================== # * New Def: Zoom * #------------------------------------------------------------------------------ # Returns the magnification ratio #============================================================================== def zoom n = (1.00 + SceneManager.scene.camera.z / 512.00) * ((@origin_y - 304) / 256.00 + 1) return n end
end #class game_enemy
#============================================================================== # ■ Game_Actor #==============================================================================
class Game_Actor < Game_Battler #============================================================================== # * Accessors * #============================================================================== attr_accessor :origin_x, :origin_y alias oc_game_actor_initialize_23kls initialize def initialize(actor_id) oc_game_actor_initialize_23kls(actor_id) @origin_x = 0 @origin_y = 0 end end #class game_actor
#============================================================================== # ■ Game_Troop #==============================================================================
class Game_Troop < Game_Unit
#============================================================================== # * Overwrite: Setup * #============================================================================== def setup(troop_id) clear @troop_id = troop_id @enemies = [] troop.members.each do |member| next unless $data_enemies[member.enemy_id] enemy = Game_Enemy.new(@enemies.size, member.enemy_id) enemy.hide if member.hidden enemy.screen_x = enemy.origin_x = member.x + (Graphics.width - 544) / 2 enemy.screen_y = enemy.origin_y = member.y + (Graphics.height - 416) @enemies.push(enemy) end init_screen_tone make_unique_names end end #class game_troop
#============================================================================== # ** Sprite_Battler ** #------------------------------------------------------------------------------ # This sprite is used to display battlers. It observes an instance of the # Game_Battler class and automatically changes sprite states. #============================================================================== class Sprite_Battler < Sprite_Base
#============================================================================== # * Alias: Update * #============================================================================== alias oc_sprite_battler_update_sfdkl update def update oc_sprite_battler_update_sfdkl
return if @battler == nil || !@battler.is_a?(Game_Enemy) self.zoom_x = self.zoom_y = @battler.zoom end end #class sprite_battler
#============================================================================== # ■ Spriteset_Battle #==============================================================================
class Spriteset_Battle #============================================================================== # * Alias: Update * #============================================================================== alias oc_spriteset_battle_update_pkwer update def update oc_spriteset_battle_update_pkwer cx, cy, cz = SceneManager.scene.camera.x, SceneManager.scene.camera.y, SceneManager.scene.camera.z bx, by = @back2_sprite.x + Graphics.width / 2, @back2_sprite.y + 304 if bx != cx || by != cy || @bz != cz # Adjust zoom if Graphics.width > 544 && Graphics.height > 416 zoom = cz / 1024.00 + 1 else zoom = cz / 416.00 + 1 end @back1_sprite.zoom_x = @back2_sprite.zoom_x = zoom * 2.0 @back1_sprite.zoom_y = @back2_sprite.zoom_y = zoom * 2.0 # Adjust coordinates @back1_sprite.x = -cx * zoom / 2 - Graphics.width / 2 + @back1_sprite.ox * 2 @back1_sprite.y = -cy * zoom / 2 - Graphics.height / 3 + @back1_sprite.oy * 2 @back2_sprite.x = -cx * zoom / 2 - Graphics.width / 2 + @back2_sprite.ox * 2 @back2_sprite.y = -cy * zoom / 2 - Graphics.height / 3 + @back2_sprite.oy * 2 @bz = cz end # Adjusts sprites' coordinates based on position relative to camera @enemy_sprites.each { |e| e.x -= SceneManager.scene.camera.x * e.battler.zoom} @enemy_sprites.each { |e| e.y -= SceneManager.scene.camera.y * e.battler.zoom} if $imported[:ve_actor_battlers] || OC::OBC::SIDEVIEW_SYSTEM @actor_sprites.each { |e| e.x -= SceneManager.scene.camera.x} @actor_sprites.each { |e| e.y -= SceneManager.scene.camera.y} if OC::OBC::SBS_ACTOR_SPRITE_Y end end end #class spriteset_battle
#============================================================================== # ■ New Class: Camera #------------------------------------------------------------------------------ # Handles the movable battle camera #==============================================================================
class Camera #============================================================================== # * Accessors * #============================================================================== attr_reader :x, :y, :z attr_accessor :camera_speed
#============================================================================== # * Initialize * #============================================================================== def initialize @x, @y, @z = 0, 0, 0 @camera_speed = OC::OBC::CAMERA_SPEED_INIT @move_x, @move_y, @move_z = 0, 0, 0 end
#============================================================================== # * Move * #------------------------------------------------------------------------------ # Repositions the battle camera #============================================================================== def move(x, y, z) @move_x = x - @x - Graphics.width / 2 @move_y = y - @y - Graphics.height / 3 @move_z = z - @z end
#============================================================================== # * Move_Target * #------------------------------------------------------------------------------ # Positions the camera to focus on an enemy #============================================================================== def move_target(target) return if target == nil && !target.is_a?(Game_Enemy) target_x = target.origin_x target_y = target.origin_y - 144 target_z = (304 - target.origin_y) * 4 move(target_x, target_y, target_z) end
#============================================================================== # * Move_Center * #------------------------------------------------------------------------------ # Positions the camera to the center #============================================================================== def move_center @move_x = -@x @move_y = -@y @move_z = -@z end
#============================================================================== # * Update * #============================================================================== def update # X-coordinate movement mv = [[@move_x.abs * @camera_speed / 160, 1].max, @camera_speed].min if @move_x > 0 @x += mv @move_x = [@move_x - mv, 0].max elsif @move_x < 0 @x -= mv @move_x = [@move_x + mv, 0].min end # Y-coordinate movement mv = [[@move_y.abs * @camera_speed / 160, 1].max, @camera_speed].min if @move_y > 0 @y += mv @move_y = [@move_y - mv, 0].max elsif @move_y < 0 @y -= mv @move_y = [@move_y + mv, 0].min end # Z-coordinate movement mv = [[@move_z.abs * @camera_speed / 96, 1].max, @camera_speed * 2].min if @move_z > 0 @z += mv @move_z = [@move_z - mv, 0].max elsif @move_z < 0 @z -= mv @move_z = [@move_z + mv, 0].min end end end #class camera
#============================================================================== # ■ BattleManager #==============================================================================
class << BattleManager #-------------------------------------------------------------------------- # * Alias method: init_members #-------------------------------------------------------------------------- alias oc_battlemanager_init_members_9jsla init_members def init_members oc_battlemanager_init_members_9jsla if $imported[:ve_actor_battlers] || OC::OBC::SIDEVIEW_SYSTEM $game_party.members.each do |member| member.origin_x = member.screen_x member.origin_y = member.screen_y end end end end #class_battlemanager
#============================================================================== # ■ Scene_Battle #==============================================================================
class Scene_Battle < Scene_Base
#============================================================================== # * Accessors * #============================================================================== attr_reader :camera #============================================================================== # * Alias: Start * #============================================================================== alias oc_scene_battle_start_ojmwe start def start @camera = Camera.new oc_scene_battle_start_ojmwe end #============================================================================== # * Alias: Update_Basic * #============================================================================== alias oc_scene_battle_update_m32ls update_basic def update_basic @camera.update oc_scene_battle_update_m32ls if @subject.is_a?(Game_Enemy) && $imported[:ve_actor_battlers] == nil && OC::OBC::SIDEVIEW_SYSTEM == false # If the target is an enemy, camera will zoom on it @camera.move_target(@subject) else # If enemy selection window is up, camera will zoom on target return if @enemy_window.nil? if @enemy_window.active if $imported["YEA-BattleEngine"] if @enemy_window.select_all? @camera.move_center else @camera.move_target(@enemy_window.enemy) end else @camera.move_target(@enemy_window.enemy) end else # If enemy selection window is not up, camera will zoom out @camera.move(Graphics.width / 2, Graphics.height / 3, -48) end end end end #class scene_battle
Script original : Rm XP (semble fonctionner avec le SBS de Minkoff) Site : lien - Code:
-
module KGC $game_special_elements = {} $imported = {} $data_states = load_data("Data/States.rxdata") $data_system = load_data("Data/System.rxdata") end
#_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/ #_/???????? - KGC_BattleCamera? #_/---------------------------------------------------------------------------- #_/?????????????????? #_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/_/
#============================================================================== # ? ???????? ? #==============================================================================
module KGC # ??????????? BC_SPEED_INIT = 24 end
#???????????????????????????????????????
$imported["BattleCamera"] = true $imported["Base Reinforce"] = true
#???????????????????????????????????????
#============================================================================== # ? Game_Enemy #==============================================================================
class Game_Enemy < Game_Battler attr_reader :origin_x, :origin_y #-------------------------------------------------------------------------- # ? ????????? #-------------------------------------------------------------------------- alias initialize_KGC_BattleCamera initialize def initialize(troop_id, member_index) # ??????? initialize_KGC_BattleCamera(troop_id, member_index)
@origin_x = $data_troops[@troop_id].members[@member_index].x @origin_y = $data_troops[@troop_id].members[@member_index].y end #-------------------------------------------------------------------------- # ? ????? X ????? #-------------------------------------------------------------------------- def screen_x return @origin_x - $scene.camera.x * self.zoom end #-------------------------------------------------------------------------- # ? ????? Y ????? #-------------------------------------------------------------------------- def screen_y return @origin_y - $scene.camera.y * self.zoom end #-------------------------------------------------------------------------- # ? ????? #-------------------------------------------------------------------------- def zoom if $game_variables[25] == 0 n = (1.00 + $scene.camera.z / 512.00) * ((@origin_y - 304) / 256.00 + 1) else n = 1 end return n end end
#???????????????????????????????????????
#============================================================================== # ? Sprite_Battler #==============================================================================
class Sprite_Battler < RPG::Sprite #-------------------------------------------------------------------------- # ? ?????? #-------------------------------------------------------------------------- alias update_KGC_BattleCamera update def update # ??????? update_KGC_BattleCamera return if @battler == nil if $game_variables[25] == 0 if @battler.is_a?(Game_Enemy) # ???? self.zoom_x = self.zoom_y = @battler.zoom end end end end
#???????????????????????????????????????
#============================================================================== # ? Spriteset_Battle #==============================================================================
class Spriteset_Battle #-------------------------------------------------------------------------- # ? ?? #-------------------------------------------------------------------------- alias update_KGC_BattleCamera update def update # ??????? update_KGC_BattleCamera
if $DEBUG if Input::trigger?(Input::Z) c = $scene.camera zoom = c.z / 512.00 + 1 p "#{c.x}, #{c.y}, #{c.z}" p "#{@battleback_sprite.x + 320}, #{@battleback_sprite.y + 304}, #{zoom}" end end # ??????? if $game_variables[25] == 0 cx, cy, cz = $scene.camera.x, $scene.camera.y, $scene.camera.z bx, by = @battleback_sprite.x + 320, @battleback_sprite.y + 304 if bx != cx || by != cy || @bz != cz # ???? zoom = cz / 512.00 + 1 @battleback_sprite.zoom_x = zoom * 1.5 @battleback_sprite.zoom_y = zoom * 1.5 # ????? if $imported["Base Reinforce"]# && KGC::BR_BATTLEBACK_FULL @battleback_sprite.ox = @battleback_sprite.bitmap.width * 0.52 # X OFFSET! @battleback_sprite.oy = @battleback_sprite.bitmap.height / 2 mag_x = 600.0 / @battleback_sprite.bitmap.width mag_y = 300.0 / @battleback_sprite.bitmap.height @battleback_sprite.zoom_x *= mag_x @battleback_sprite.zoom_y *= mag_y end # ???? @battleback_sprite.x = -cx * zoom / 2 - 320 + @battleback_sprite.ox * 2 @battleback_sprite.y = -cy * zoom / 2 - 144 + @battleback_sprite.oy * 2 @bz = cz end end end end
#???????????????????????????????????????
#============================================================================== # ? Camera #------------------------------------------------------------------------------ # ????????????????? #==============================================================================
class Camera #-------------------------------------------------------------------------- # ? ?????????? #-------------------------------------------------------------------------- attr_reader :x, :y, :z attr_accessor :move_speed #-------------------------------------------------------------------------- # ? ????????? #-------------------------------------------------------------------------- def initialize @x, @y, @z = 0, 0, 0 @move_x = @move_y = @move_z = 0 @move_speed = KGC::BC_SPEED_INIT end #-------------------------------------------------------------------------- # ? ?? #-------------------------------------------------------------------------- def move(x, y, z) @move_x, @move_y, @move_z = x - @x - 320, y - @y - 160, z - @z end #-------------------------------------------------------------------------- # ? ????????? #-------------------------------------------------------------------------- def move_target(target) return if target == nil || !target.is_a?(Game_Enemy) if $game_variables[25] == 0 tx, ty = target.origin_x, target.origin_y - 144 tz = (304 - target.origin_y) * 5 move(tx, ty, tz) end end #-------------------------------------------------------------------------- # ? ????? #-------------------------------------------------------------------------- def centering @move_x, @move_y, @move_z = -@x, -@y, -@z end #-------------------------------------------------------------------------- # ? ?? #-------------------------------------------------------------------------- def update # X???? if $game_variables[25] == 0 mv = [[@move_x.abs * @move_speed / 160, 1].max, @move_speed].min if @move_x > 0 @x += mv @move_x = [@move_x - mv, 0].max elsif @move_x < 0 @x -= mv @move_x = [@move_x + mv, 0].min end # Y???? mv = [[@move_y.abs * @move_speed / 160, 1].max, @move_speed].min if @move_y > 0 @y += mv @move_y = [@move_y - mv, 0].max elsif @move_y < 0 @y -= mv @move_y = [@move_y + mv, 0].min end # Z???? mv = [[@move_z.abs * @move_speed / 96, 1].max, @move_speed * 2].min if @move_z > 0 @z += mv @move_z = [@move_z - mv, 0].max elsif @move_z < 0 @z -= mv @move_z = [@move_z + mv, 0].min end end end end
#???????????????????????????????????????
#============================================================================== # ? Scene_Battle (???? 1) #==============================================================================
class Scene_Battle attr_reader :camera #-------------------------------------------------------------------------- # ? ????? #-------------------------------------------------------------------------- alias main_KGC_BattleCamera main def main # ????? @camera = Camera.new # ??????? main_KGC_BattleCamera end #-------------------------------------------------------------------------- # ? ?????? #-------------------------------------------------------------------------- alias update_KGC_BattleCamera update def update @camera.update
# ??????? update_KGC_BattleCamera end end
#???????????????????????????????????????
#============================================================================== # ? Scene_Battle (???? 3) #==============================================================================
class Scene_Battle #-------------------------------------------------------------------------- # ? ?????? (???????????? : ??????) #-------------------------------------------------------------------------- alias update_phase3_enemy_select_KGC_BattleCamera update_phase3_enemy_select def update_phase3_enemy_select # ??????????? if $game_variables[25] == 0 if !$imported["ActiveCountBattle"] || @action_battler == nil @camera.move_target(@enemy_arrow.enemy) end end # ??????? update_phase3_enemy_select_KGC_BattleCamera end #-------------------------------------------------------------------------- # ? ???????? #-------------------------------------------------------------------------- alias end_enemy_select_KGC_BattleCamera end_enemy_select def end_enemy_select # ????????? if $game_variables[25] == 0 if !$imported["ActiveCountBattle"] || @action_battler == nil @camera.move(320, 160, 0) end end # ??????? end_enemy_select_KGC_BattleCamera end end #???????????????????????????????????????
#============================================================================== # ? Scene_Battle (???? 4) #==============================================================================
class Scene_Battle #-------------------------------------------------------------------------- # ? ?????? (??????? ???? 3 : ??????????) #-------------------------------------------------------------------------- alias update_phase4_step3_KGC_BattleCamera update_phase4_step3 def update_phase4_step3 # ????? if $game_variables[25] == 0 if @active_battler.is_a?(Game_Actor) && @target_battlers != [] if @target_battlers.size > 1 @camera.move(320, 160, -96) else @camera.move_target(@target_battlers[0]) end elsif @active_battler.is_a?(Game_Enemy) @camera.move_target(@active_battler) end end # ??????? update_phase4_step3_KGC_BattleCamera end
#-------------------------------------------------------------------------- # ? ?????? (??????? ???? 6 : ??????) #-------------------------------------------------------------------------- alias update_phase4_step6_KGC_BattleCamera update_phase4_step6 def update_phase4_step6 # ??????????? @camera.centering
# ??????? update_phase4_step6_KGC_BattleCamera end end
Dernière édition par Chaos17 le Sam 2 Mar 2013 - 16:41, édité 5 fois |
|
Invité Invité

![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 0:11 | |
| Omg tu n'aurais pas le même pour VX ? =/ Merci du partage je t'ajoute des points.  |
|
Likali Ancien staffeux
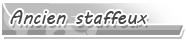

 Messages postés : 1905 Date d'inscription : 14/10/2012 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 0:16 | |
| Fait attention ! Il y a des smileys dans le code XD Très bon partage, merci  |
|
knighty Chevalier (niveau 5)


 Messages postés : 133 Date d'inscription : 30/01/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 0:20 | |
| Si il est compatible avec les scripts de combat de Moghunter pour VX Ace, alors il y a des chances que je l'utilise. J'ai toujours bien aimé les effets de caméra, ça donne un certain dynamisme. Merci . |
|
Zexion Administrateur

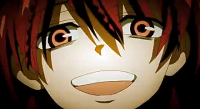
 Messages postés : 6228 Date d'inscription : 04/01/2012 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 0:29 | |
| - Likali a écrit:
- Fait attention ! Il y a des smileys dans le code XD
Réparé. Merci pour le signalement. |
|
Chaos17 Chevalier (niveau 2)
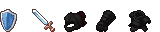

 Messages postés : 90 Date d'inscription : 09/02/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 4:28 | |
| Merci d'avoir réparer le script, j'utiliserai à l'avenir la fonction code. - Citation :
- Omg tu n'aurais pas le même pour VX ? =/
Non malheureusement, la version original du script est la base pour Rpg maker Xp. Je vais la mettre dans mon premier poste, parce qu'elle risque de disparaitre. - knighty a écrit:
- Si il est compatible avec les scripts de combat de Moghunter pour VX Ace, alors il y a des chances que je l'utilise. J'ai toujours bien aimé les effets de caméra, ça donne un certain dynamisme. Merci .
Possibilité de problème avec le battler motion de Moghunter comme dans mon SBS, mais peut-être que tu n'auras pas de soucis si tu utilises la vue par défaut car il me semble que Moghuter n'y change rien. |
|
knighty Chevalier (niveau 5)


 Messages postés : 133 Date d'inscription : 30/01/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 12:26 | |
| J'utilise la vue par défaut, donc ça devrait pas être un problème. Merci. |
|
Invité Invité

![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 13:41 | |
| Bon bah tant pis. Tu as rajouté la version XP, ça mérite donc quelques points. ^^ |
|
Jin Ancien staffeux
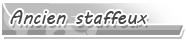

 Messages postés : 8557 Date d'inscription : 08/12/2010 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 15:14 | |
| Sur un combat de vu coté, je ne sais pas si le rendu est le même ... Si c'est un combat vu de dos je dis pas, mais de coté ca doit pas être super ^^
Merci du partage ne tout cas =) |
|
Chaos17 Chevalier (niveau 2)
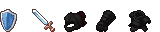

 Messages postés : 90 Date d'inscription : 09/02/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 16:42 | |
| Mise à jour du script (voir premier post). L'auteur a fait en sorte que ça ne bug plus avec les SBS : testé avec JET. Ne fonctionne toujours pas à le script de motion battle de Moghunter.
Dernière édition par Chaos17 le Sam 2 Mar 2013 - 18:16, édité 1 fois |
|
Jin Ancien staffeux
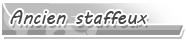

 Messages postés : 8557 Date d'inscription : 08/12/2010 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 17:41 | |
| En même temps moghunter code pas super bien. Il a de bon script mais bon, tout ses scripts créent des incompatibilités ^^ |
|
Chaos17 Chevalier (niveau 2)
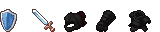

 Messages postés : 90 Date d'inscription : 09/02/2013 Jauge LPC :
![[XP - ACE] Battle camera Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [XP - ACE] Battle camera Sam 2 Mar 2013 - 18:19 | |
| Je ne suis qu'un utilisateur lambda, je ne juge pas sur le code mais sur la compatibilité des scripts et leur facilité d'utilisation. J'apprécie les scripts de Moghunter. |
|