|
| Auteur | Message |
---|
axel4 Ancien staffeux
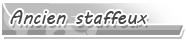

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: [VX] Difficulté adaptée Lun 1 Sep 2008 - 14:08 | |
| Pour une difficulté adaptée a votre personnage.
Je sais pas si il marche^^automatiquement.
#============================================================================== # ** Dynamic Difficulty #------------------------------------------------------------------------------ # By: DrakoShade # Version 1.1 # Last Updated: 17 March, 2008 #------------------------------------------------------------------------------ # Version History: # V. 1.1: Updated the note-parsing methodology to work as described by "loam" # of RMXP.org. This script's use of the Note field will no longer # interfere with or be confused by other notes. Also, pointless # methods for the difficulty setting have been removed. #============================================================================== =begin Here, you get a chance to set the defaults for your creatures. Any creature for which the Note tab doesn't include a modification will use the numbers you set here. Instructions follow. =end module RPG class Enemy def get_default_gains @maxhp_gain = 50 #Default 50 @maxmp_gain = 10 #Default 10 @atk_gain = 1.25 #Default 1.25 @def_gain = 1.25 #Default 1.25 @spi_gain = 2.5 #Default 2.5 @agi_gain = 2.5 #Default 2.5 @hit_gain = 0 #Default 0 @eva_gain = 0 #Default 0 @exp_gain = 0 #Default 0 @gold_gain = 0 #Default 0 @variance = 15 #Default 15 @min_level = 1 #Default 1 @max_level = 99 #Default 99 end end end
=begin ======================================================================== || Using the Note Field to your advantage. || =============================================================================== The defaults that you set above are fine if you want every single enemy in the game to follow the same growth pattern. If that's the case, you don't have to use the Note field at all for this script. If you want your enemies to grow in different ways from one another, then the Note field is where you make it happen.
To do anything in the Notes with this script, you will need to include two special lines in the box: "=begin dynamic difficulty" "=end dynamic difficulty" Don't put quotes around them. Those are simply here so that this explanation won't mess with the commenting in the script itself. Anything that falls between those two lines will be evaluated just after the individual enemy looks up the defaults above. Thus, if you include
@gold_gain = 200
then that specific enemy will be worth an extra 200 gold for every level it gains, no matter what default you have set. If, instead, you use the line
@gold_gain *= 2
then the enemy is worth twice the gold-per-level of your standard. If the standard stays at 0, of course, then it's still worth 0 a level, but you know what I mean.
=============================================================================== || The Minimum and Maximum Levels || =============================================================================== In the defaults, you may have noticed the defaults for @min_level and @max_level. A creature for which @min_level = 1 and @max_level = 99 will gain power as long as your party's average level is above 1, and won't stop gaining power until level 99, which can't normally be exceeded anyway. This works well if you intend the enemy to scale no matter what level it's encountered at, but you can do it differently.
An enemy for which @min_level = 10, for example, will be at the power you set in the database any time it's encountered by a party of level 10 or lower. When said party hits level 11, that enemy will gain only 1 level, rather than the 10 that anything else would gain. In this way, you can create enemies that are never incredibly weak, but still get stronger.
@max_level is similar, but for the other end of the spectrum. An enemy for which @max_level = 30, for instance, would scale as normal up until the party reached that level. but if the party were level 40, it would still only grow as if the party were level 30. Thus, this enemy stops gaining power when the party's average level hits it's @max_level.
=============================================================================== || Game Difficulty || =============================================================================== At any point, you can set the value of the game's difficulty with a simple script call. Since it's an attr_accessor, all you have to do is one of these: $game_system.difficulty = x $game_system.difficulty += x $game_system.difficulty -= x Etcetera, so on, and so forth.
This script uses the difficulty to determine the level of the enemies, mostly like the party's average level, but with a small difference. When setting how powerful a monster is, for its maximum HP, attack and defense, etc. the script will add the difficulty to the party's level. However, when determining the rewards of experience and gold returns, the script ignores this value.
Example: An enemy at level 8 and game difficulty of 2 will be just as hard to beat as the same enemy at level 10 and game difficulty 0, but will give rewards the same as if you'd beat it at level 8 and difficulty 0.
=============================================================================== || General Advice || =============================================================================== When designing your enemies, take into account the level at which you want them to be a real challenge for your party. Their stats should be roughly similar to those of a party member at their @min_level wearing the equipment you'd expect your party to have at the target level.
In this way, although the enemy scales down to match a party whose level is lower than expected, he still fights as if he were equipped to take on the stronger party. The ability to defeat him will, at this point, hinge more on your party's equipment than their level. Of course, the act of balancing is purely up to you. My default numbers are set so that enemies will scale with a new actor's default gains in the database. You could retool them to scale with equipment as well, or factor how well-equipped your enemy is into his stats at minimum level. The choice is yours.
I hope you enjoy using this script. Happy creating.
=============================================================================== || That's it. On to the rest of the script. || =============================================================================== =end #============================================================================== # ** Game_System #------------------------------------------------------------------------------ # The actual difficulty of the game as a whole is added into this class. #============================================================================== class Game_System attr_accessor :difficulty #New public instance variable. #---------------------------------------------------------------------------- # * Aliasing the Initialize method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_initialize initialize def initialize drakoshade_dynamic_difficulty_initialize @difficulty = 0 end end #============================================================================== # End modifications to Game_System. #==============================================================================
#============================================================================== # ** RPG::Enemies #------------------------------------------------------------------------------ # Most of the magic happens here. New methods are created which allow the # enemies to gain levels, based on a combination of both the party's average # level and the new $game_system.difficulty. #============================================================================== module RPG class Enemy #-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_accessor :base_maxhp, :base_maxmp, :base_atk, :base_def, :base_spi attr_accessor :base_agi, :base_hit, :base_eva, :base_exp, :base_gold attr_accessor :maxhp_gain, :maxmp_gain, :atk_gain, :def_gain, :spi_gain attr_accessor :agi_gain, :hit_gain, :eva_gain, :exp_gain, :gold_gain attr_accessor :min_level, :max_level #-------------------------------------------------------------------------- # * Set_Bases #-------------------------------------------------------------------------- def set_bases @base_maxhp, @base_maxmp = @maxhp, @maxmp @base_atk, @base_def = @atk, @def @base_spi, @base_agi = @spi, @agi @base_hit, @base_eva = @hit, @eva @base_exp, @base_gold = @exp, @gold get_default_gains eval(@note[/(?<==begin dynamic difficulty)(.*?)(?==end dynamic difficulty)/m].to_s) end #-------------------------------------------------------------------------- # * Update Level #-------------------------------------------------------------------------- def update_level levels = [([$game_party.ave_level, @max_level].min - @min_level), 0].max diff = $game_system.difficulty @maxhp = generate_stat(@base_maxhp-1, @maxhp_gain, (levels + diff)) + 1 @maxmp = generate_stat(@base_maxmp-1, @maxmp_gain, (levels + diff)) + 1 @atk = generate_stat(@base_atk-1, @atk_gain, (levels + diff)) + 1 @def = generate_stat(@base_def-1, @def_gain, (levels + diff)) + 1 @spi = generate_stat(@base_spi-1, @spi_gain, (levels + diff)) + 1 @agi = generate_stat(@base_agi-1, @agi_gain, (levels + diff)) + 1 @hit = generate_stat(@base_agi, @agi_gain, (levels + diff)) @eva = generate_stat(@base_eva, @eva_gain, (levels + diff)) @exp = generate_stat(@base_exp, @exp_gain, levels) @gold = generate_stat(@base_gold, @gold_gain, levels) end #-------------------------------------------------------------------------- # * Generate Stat #-------------------------------------------------------------------------- def generate_stat(base, gain, levels) result = base + (gain * levels) result *= (rand(@variance*2)+(100-@variance)) result /= 100 result = [result.to_i, 0].max return result end end end
#============================================================================== # ** Game_Party #------------------------------------------------------------------------------ # I'm adding a method here that will allow this script (or any other) to # determine the average party level. #============================================================================== class Game_Party def ave_level level = 0 for i in @actors actor = $game_actors[i] level += actor.level end level /= @actors.size return level.round end end
#============================================================================== # ** Scene_Title #------------------------------------------------------------------------------ # Methods that load the database need to be altered so that the enemies set # their bases. #============================================================================== class Scene_Title #---------------------------------------------------------------------------- # *Alias the Load Database method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_load_database load_database def load_database drakoshade_dynamic_difficulty_load_database for i in 1...$data_enemies.size $data_enemies[i].set_bases end end #---------------------------------------------------------------------------- # *Alias the Load Battle Test Database method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_load_bt_database load_bt_database def load_bt_database drakoshade_dynamic_difficulty_load_bt_database for i in 1...$data_enemies.size $data_enemies[i].set_bases end end end
#============================================================================== # ** Game_Troop #------------------------------------------------------------------------------ # The modification here allows for greater versitility than modifying the # battle scene in this situation. Enemies will load into the troop with # updated stats. #============================================================================== class Game_Troop #---------------------------------------------------------------------------- # *Alias the Setup method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_setup setup def setup(troop_id) for i in 1...$data_enemies.size $data_enemies[i].update_level end drakoshade_dynamic_difficulty_setup(troop_id) end end |
|  | | Elekami Fondateur


 Messages postés : 19071 Date d'inscription : 19/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mar 2 Sep 2008 - 13:04 | |
| Y a pas d'explication pour l'utilisation? |
|  | | axel4 Ancien staffeux
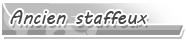

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mar 2 Sep 2008 - 14:53 | |
| Non désolé je l'ai trouvé je crois qu'il marche automatiquement si tu veux je peut faire une recherche |
|  | | Namor41 Ancien staffeux
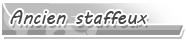

 Messages postés : 721 Date d'inscription : 23/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mar 2 Sep 2008 - 18:16 | |
| Oui, il y a des explication mais elles sont en anglais. Je les traduit si vous voulez et je remet le script avec les explications traduite en français.
P.S.: Si vous voulez je peux traduire tout se qui est en anglais, peut importe quoi. Enfin il me faudra une demande clair et précise au préalable. |
|  | | axel4 Ancien staffeux
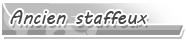

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mar 2 Sep 2008 - 20:58 | |
| Bin OUI sa sera beaucoup mieux merci de proposer^^ |
|  | | Namor41 Ancien staffeux
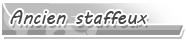

 Messages postés : 721 Date d'inscription : 23/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Traduction de l'en-tête du script... Mer 3 Sep 2008 - 5:53 | |
| Bonjour, Voilà l'en-tête du script traduit en français: - Spoiler:
#============================================================================== # ** Dynamic Difficulty #------------------------------------------------------------------------------ # By: DrakoShade # Version 1.1 # Last Updated: 17 March, 2008 #------------------------------------------------------------------------------ # Version History: # V. 1.1: Updated the note-parsing methodology to work as described by "loam" # of RMXP.org. This script's use of the Note field will no longer # interfere with or be confused by other notes. Also, pointless # methods for the difficulty setting have been removed. #============================================================================== =begin Ici, vous avez la chance de sécler les paramètres par défaut pour vos créatures. N'importe quelle créature pour laquelle la table de Note n'inclu pas une modifi- tion, utilisera les numéros que vous séclerez ici. Les instructions suivent. =end module RPG class Enemy def get_default_gains @maxhp_gain = 50 #Default 50 @maxmp_gain = 10 #Default 10 @atk_gain = 1.25 #Default 1.25 @def_gain = 1.25 #Default 1.25 @spi_gain = 2.5 #Default 2.5 @agi_gain = 2.5 #Default 2.5 @hit_gain = 0 #Default 0 @eva_gain = 0 #Default 0 @exp_gain = 0 #Default 0 @gold_gain = 0 #Default 0 @variance = 15 #Default 15 @min_level = 1 #Default 1 @max_level = 99 #Default 99 end end end
=begin ======================================================================== || Utilisez le champ de Note à votre avantage. || =============================================================================== Les paramètres par défaut que vous avez séclez au-dessus seront correct pour chaque ennemi dans le jeu qui suivront le même patron de croissance. Si c'est le cas, vous n'aurez pas à utiliser le champ de Note pour se script. Si vous voulez que vos ennemis croissent d'une façon différente, alors le champ de Note est là où vous en aurez besoin. Pour faire quelque chose avec se script, vous aurez besoin d'inclure 2 lignes spéciales dans la boite: "=begin dynamic difficulty" "end dunamic difficulty" Ne mettez pas les guillemets. Ils sont simplement ici pour que l'explication ne se mêle pas avec les commentaires dans le script lui-même. Tout se qui tombera entre ses 2 lignes sera évalué juste après que l'ennemi individuel regarde en haut sur les paramètres par défaut ci-haut. Par contre, si vous incluez:
@gold_gain = 200
alors cet ennemi spécifique donnera un extra de 200 piéce d'or pour chaque niveau qu'il prendra, peut importe les paramètres par défaut que vous avez séclé. Si, à la place vous utilisez cette ligne:
@gold_gain *= 2
alors l'ennemi donnera 2 fois le nombre de pièce d'or-par niveau de votre standart. Si le standart reste à zéro, alors cela donnera zéro par niveau, mais vous savez se que je veux dire.
=============================================================================== || Le niveau Minimum et Maximum || =============================================================================== Dans les paramètres par défaut, vous avez sans doute notez les paramètres pour le @min_level et le @max_level. Une créature qui a un @min_level = 1 et que le @max_level = 99 obtiendra de la force aussi longtemps que votre équipe sera en moyenne à un niveau au-dessus de 1 et il n'arrêtera pas d'en prendre jusqu'au niveau 99, qui ne peut être dépasser de toute façon. Ceci fonctionnera peut importe à quelle échelle de niveau où vous le rencontrer, mais vous pouvez en décidez autrement. Un ennemi qui à pour @min_level = 10, par exemple, sera à la force que vous aurez séclez dans la base de donnée à chaque fois qu'il rencontrera une équipe au même niveau ou plus bas. Quant l'équipe atteindra le niveau 11, l'ennemi ne gagnera qu'un seul niveau. De cette façon, vous créerez des ennemis qui ne seront jamais incroyablement faible, mais pourront devenir plus fort. Le @max_level est similaire, mais il est de l'autre côté du spectre. Un ennemi qui aura un @max_level = 30, par exemple, montera normalement jusqu'à se que l'équipe atteigne se niveau. Mais, si votre équipe est au niveau 40, il ne sera pas plus fort que si votre équipe en serait qu'au niveau 30. Cet ennemi cessera donc de gagner de la force quant l'éqipe atteindra une moyenne de niveau de son @max_level.
=============================================================================== || Difficulté du jeu || =============================================================================== A chaque moment vous pouvez cécler la valeur de la difficulté du jeu avec un simple appel de script. Puisque c'est un attr_accessor, tout se que vous avez à faire est l'une de ses commandes: $game_system.difficulty = x $game_system.difficulty += x $game_system.difficulty -= x Etc...
Ce script utilise les difficultés pour déterminer le niveau des ennemis, le plus souvent comme le niveau moyen de votre équipe, mais avec une petite différence. Quant vous séclez comment le monstre sera fort, pour son HP max, son attaque, sa défense, etc. le script ejoutera la difficulté à la hauteur du niveau de votre équipe. Par contre, quant vous determinez les récompenses et l'or, le script ignorera ces valeurs.
Exemple: Un ennemi au niveau 8 et une difficulté de jeu à 2, sera plus dure à vaincre que le même ennemi au niveau 10 avec une difficulté de jeu à zéro, mais ils donneront les mêmes récompenses que si vous le battez au niveau 8 avec la difficulté à zéro.
=============================================================================== || Conseil Général.|| =============================================================================== Quant vous créez vos ennemis, prenez en compte le niveau qui pourra être un bon challenge pour votre équipe. Leurs statistiques devront être similaire à ceux de votre équipe à leur @min_level en ayant l'équippement que vous planifirez pour votre équipe qu'elle aura à se niveau spécifique.
Dans cette optique, même si l'ennemi est à une échelle inférieure à votre équipe que vous l'avez planifiez, il pourra se battre comme si il était équipé pour battre une équipe plus forte. L'habileté à le défier, sera, à se point, plus accez sur l'équippement de votre équipe que sur son niveau. Bien sûr, l'acte de balancement est purement à votre guise. Vous pouvez adapter les chiffres comme vous le souhaité.
J'espère que vous aimerez utilisez se script. Bonne création.
=============================================================================== || C'est tout. Voici le reste du script. ||
=============================================================================== =end #============================================================================== # ** Game_System #------------------------------------------------------------------------------ # The actual difficulty of the game as a whole is added into this class. #============================================================================== class Game_System attr_accessor :difficulty #New public instance variable.
#---------------------------------------------------------------------------- # * Aliasing the Initialize method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_initialize initialize def initialize drakoshade_dynamic_difficulty_initialize @difficulty = 0 end end #============================================================================== # End modifications to Game_System. #==============================================================================
#============================================================================== # ** RPG::Enemies #------------------------------------------------------------------------------ # Most of the magic happens here. New methods are created which allow the # enemies to gain levels, based on a combination of both the party's average # level and the new $game_system.difficulty. #============================================================================== module RPG class Enemy #-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_accessor :base_maxhp, :base_maxmp, :base_atk, :base_def, :base_spi attr_accessor :base_agi, :base_hit, :base_eva, :base_exp, :base_gold attr_accessor :maxhp_gain, :maxmp_gain, :atk_gain, :def_gain, :spi_gain attr_accessor :agi_gain, :hit_gain, :eva_gain, :exp_gain, :gold_gain attr_accessor :min_level, :max_level
#-------------------------------------------------------------------------- # * Set_Bases #-------------------------------------------------------------------------- def set_bases @base_maxhp, @base_maxmp = @maxhp, @maxmp @base_atk, @base_def = @atk, @def @base_spi, @base_agi = @spi, @agi @base_hit, @base_eva = @hit, @eva @base_exp, @base_gold = @exp, @gold get_default_gains eval(@note[/(?<==begin dynamic difficulty)(.*?)(?==end dynamic difficulty)/m].to_s) end
#-------------------------------------------------------------------------- # * Update Level #-------------------------------------------------------------------------- def update_level levels = [([$game_party.ave_level, @max_level].min - @min_level), 0].max diff = $game_system.difficulty @maxhp = generate_stat(@base_maxhp-1, @maxhp_gain, (levels + diff)) + 1 @maxmp = generate_stat(@base_maxmp-1, @maxmp_gain, (levels + diff)) + 1 @atk = generate_stat(@base_atk-1, @atk_gain, (levels + diff)) + 1 @def = generate_stat(@base_def-1, @def_gain, (levels + diff)) + 1 @spi = generate_stat(@base_spi-1, @spi_gain, (levels + diff)) + 1 @agi = generate_stat(@base_agi-1, @agi_gain, (levels + diff)) + 1 @hit = generate_stat(@base_agi, @agi_gain, (levels + diff)) @eva = generate_stat(@base_eva, @eva_gain, (levels + diff)) @exp = generate_stat(@base_exp, @exp_gain, levels) @gold = generate_stat(@base_gold, @gold_gain, levels) end
#-------------------------------------------------------------------------- # * Generate Stat #-------------------------------------------------------------------------- def generate_stat(base, gain, levels) result = base + (gain * levels) result *= (rand(@variance*2)+(100-@variance)) result /= 100 result = [result.to_i, 0].max return result end end end
#============================================================================== # ** Game_Party #------------------------------------------------------------------------------ # I'm adding a method here that will allow this script (or any other) to # determine the average party level. #============================================================================== class Game_Party def ave_level level = 0 for i in @actors actor = $game_actors[i] level += actor.level end level /= @actors.size return level.round end end
#============================================================================== # ** Scene_Title #------------------------------------------------------------------------------ # Methods that load the database need to be altered so that the enemies set # their bases. #============================================================================== class Scene_Title #---------------------------------------------------------------------------- # *Alias the Load Database method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_load_database load_database def load_database drakoshade_dynamic_difficulty_load_database for i in 1...$data_enemies.size $data_enemies[i].set_bases end end
#---------------------------------------------------------------------------- # *Alias the Load Battle Test Database method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_load_bt_database load_bt_database def load_bt_database drakoshade_dynamic_difficulty_load_bt_database for i in 1...$data_enemies.size $data_enemies[i].set_bases end end end
#============================================================================== # ** Game_Troop #------------------------------------------------------------------------------ # The modification here allows for greater versitility than modifying the # battle scene in this situation. Enemies will load into the troop with # updated stats. #============================================================================== class Game_Troop #---------------------------------------------------------------------------- # *Alias the Setup method. #---------------------------------------------------------------------------- alias drakoshade_dynamic_difficulty_setup setup def setup(troop_id) for i in 1...$data_enemies.size $data_enemies[i].update_level end drakoshade_dynamic_difficulty_setup(troop_id) end end
J'espère que vous comprendrez comment il fonctionne. Je souhaite juste qu'il n'y est pas d'erreur de syntaxe. Personnellement je pourrait lui trouver une utilité sur mon projet actuel. Merci pour se script. |
|  | | axel4 Ancien staffeux
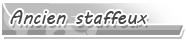

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mer 3 Sep 2008 - 14:00 | |
| De rien je suis heureux de pouvoir t'avoir aider et merci pour la version traduite mais peut tu expliquer son fonctionnement s'il te plaît. |
|  | | Namor41 Ancien staffeux
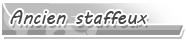

 Messages postés : 721 Date d'inscription : 23/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mer 3 Sep 2008 - 18:27 | |
| Oui, voilà comment je l'ai compris:
Au début du script tu verras plusieurs caractéristiques: maxhp_gain, maxmp_gain, atk_gain... tu peux les laisser comme sa ou tu peux modifier les chiffres par défaut pour l'adapter à ton jeu. Ces valeurs servent à déterminer de combien les ennemis monteront dans leurs caractéristiques (leur statut). Ou si tu préfère, leur évolution en niveau suivra l'évolution de tes personnages (les héros). Le niveau de difficulté du jeu peut lui-aussi être adapté à ton jeu. Tu dois par contre placé un script sur tes cartes comme suit(enfin je crois):
$game_system_difficulty = x (le x étant le chiffre pour ta difficulté.) Pour le reste regarde dans le script (se qui est en français), c'est assez bien expliqué. Je ne l'ai pas testé, donc pour l'instant je ne peux pas en dire davantage. J'espère que tu pourras comprendre mieux à quoi se script sert. Pour ma part, je dois avouer qu'il me sera très utile pour ne pas avoir des monstres trop faible. Voilà |
|  | | axel4 Ancien staffeux
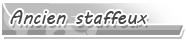

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Mer 3 Sep 2008 - 19:07 | |
| On dirai qu'il fait pas tous automatiquement :s |
|  | | Elekami Fondateur


 Messages postés : 19071 Date d'inscription : 19/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Jeu 4 Sep 2008 - 18:08 | |
| Axel4, la prochaine va un peut plus loin quand tu post un script, mais bon merci quand même de ce partage! Namor41, merci pour la traduction! |
|  | | Namor41 Ancien staffeux
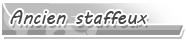

 Messages postés : 721 Date d'inscription : 23/07/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Jeu 4 Sep 2008 - 18:13 | |
| Et bien de rien, sa me fait plaisir Si il y a autre chose à traduire n'hésitez pas à me le demander. |
|  | | axel4 Ancien staffeux
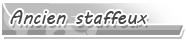

 Messages postés : 684 Date d'inscription : 31/08/2008 Jauge LPC :
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée Jeu 4 Sep 2008 - 19:39 | |
| EUH comment sa un peu plus loin  |
|  | | Contenu sponsorisé
![[VX] Difficulté adaptée Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Difficulté adaptée ![[VX] Difficulté adaptée Empty](https://2img.net/i/empty.gif) | |
| |
|  | | |
Sujets similaires |  |
|
| Permission de ce forum: | Vous ne pouvez pas répondre aux sujets dans ce forum
| |
| |
| |